How to create Scalable Programs to be a Developer By Gustavo Woltmann
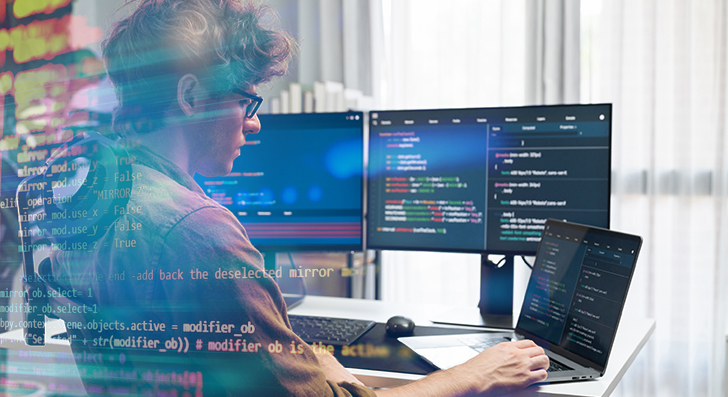
Scalability signifies your application can cope with expansion—a lot more customers, extra facts, plus much more targeted traffic—without having breaking. As being a developer, creating with scalability in your mind saves time and stress afterwards. Right here’s a transparent and useful guide to assist you to start by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Numerous apps fail if they develop rapid simply because the first style can’t cope with the extra load. To be a developer, you should Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to become flexible. Keep away from monolithic codebases where almost everything is tightly related. Alternatively, use modular structure or microservices. These patterns split your application into smaller, unbiased parts. Each and every module or company can scale on its own with no influencing the whole process.
Also, consider your database from day a single. Will it will need to handle 1,000,000 end users or simply just a hundred? Select the appropriate sort—relational or NoSQL—based upon how your details will grow. Program for sharding, indexing, and backups early, Even though you don’t have to have them yet.
Yet another significant stage is to prevent hardcoding assumptions. Don’t compose code that only will work less than current circumstances. Take into consideration what would come about When your consumer base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like information queues or celebration-pushed techniques. These enable your application cope with additional requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you're lessening upcoming complications. A properly-planned system is less complicated to take care of, adapt, and increase. It’s far better to organize early than to rebuild later.
Use the Right Databases
Selecting the correct databases is often a essential A part of building scalable purposes. Not all databases are crafted precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your app grows.
Get started by comprehension your facts. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They are potent with associations, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and facts.
Should your details is much more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and can scale horizontally additional effortlessly.
Also, look at your read and publish styles. Are you currently executing lots of reads with less writes? Use caching and browse replicas. Are you handling a major create load? Investigate databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short term facts streams).
It’s also smart to Believe ahead. You may not need to have Highly developed scaling features now, but choosing a database that supports them implies you gained’t have to have to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases performance as you grow.
In short, the proper database depends on your app’s composition, velocity requires, And exactly how you be expecting it to grow. Take time to select sensibly—it’ll help save many difficulties later on.
Optimize Code and Queries
Quick code is essential to scalability. As your application grows, just about every compact hold off adds up. Badly created code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing cleanse, basic code. Stay away from repeating logic and remove nearly anything unneeded. Don’t choose the most elaborate Option if an easy one is effective. Maintain your features short, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using an excessive amount memory.
Up coming, evaluate your database queries. These often sluggish things down a lot more than the code itself. Be sure Every question only asks for the data you really have to have. Prevent Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, Specifically throughout large tables.
In case you discover the exact same data currently being asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done fine with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of more users and much more visitors. If every thing goes by means of a single server, it's going to promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Instead of one server carrying out all of the work, the load balancer routes buyers to distinctive servers based upon availability. What this means is no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar data once more—like an item webpage or perhaps a profile—you don’t really need to fetch it through the database every time. You may serve it within the cache.
There are 2 popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static information near the user.
Caching lessens database load, enhances speed, and helps make your application a lot more economical.
Use caching for things that don’t transform often. And constantly make sure your cache is up-to-date when details does transform.
In brief, load balancing and caching are uncomplicated but effective instruments. With each other, they assist your application deal with additional customers, keep fast, and Recuperate from challenges. If you plan to expand, you require the two.
Use Cloud and Container Instruments
To build scalable programs, you may need applications that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and services as you'll need them. You don’t really need to obtain components or guess upcoming potential. When traffic raises, you'll be able to incorporate additional methods with just some clicks or quickly making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety equipment. It is possible to target constructing your app as opposed to controlling infrastructure.
Containers are Yet another important Instrument. A container offers your application and everything it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application works by using a number of containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
To put it briefly, employing cloud and container tools suggests you'll be able to scale speedy, deploy simply, and recover speedily when problems transpire. If you would like your application to grow with no limits, start off using these equipment early. They save time, minimize hazard, and assist you stay centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t observe your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a essential A part of constructing scalable devices.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it will require for people to load internet pages, how often mistakes take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for critical troubles. By way of example, When your reaction time goes previously mentioned a limit or even a support goes down, you ought to get notified quickly. This aids you resolve concerns quick, often ahead of consumers even recognize.
Monitoring is usually handy any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about actual damage.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll pass up signs of trouble until it’s far too late. But with the best tools set up, you remain on top of things.
In a nutshell, checking aids you keep your app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your program and ensuring that it works perfectly, even under pressure.
Closing Ideas
Scalability check here isn’t just for major businesses. Even smaller apps will need a strong foundation. By creating diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without breaking under pressure. Start out small, Feel major, and Develop sensible.